JavaScript Slide Show with CSS

In a previous article we created a very simple JavaScript slide show. This slide show is completely functional, and even displays something appropriate for people who don't have JavaScript, but it doesn't quite have all the features I'd like to have for my website. In particular, I'd like to have the thumbnail of the image I'm currently displaying somehow look different from the other thumbnails. Since styles are a good way to accomplish this, I am going to start by converting all of the attributes in my existing HTML to CSS.
First I converted my existing styling to use CSS. If you aren't familiar with CSS, the easiest way to start experimenting with it is to put it between style tags in the head of your HTML document. The initial tag needs a type attribute to tell the browser what type of style information you are using, so it should look like this:
The initial conversion to CSS was easy since only the large image had styling information and it already had an id attribute that was being used for JavaScript purposes.
I hadn't previously included sizing information for the thumbnails, but I added a class called thumbs and set image tags in that class to set the size to 40px by 40px. This means that even if I accidentally load images that are too large or small for thumbnails, they will be forced to display in thumbnail size.
I also added a slideshow class to hold the entire slideshow. This will allow me to do things like add a border or change the background color for the whole slideshow if I desire. At this point I'm only using it to set the maximum height to the the height of the large image, because when I add more thumbnails I want them to stay on the side of the large image instead of moving above it. Unfortunately, Internet Explorer doesn't support the max-height attribute, so I'll still have to work on this issue more later.
Finally I created a style for the selected thumbnail. I decided that I wanted my selected thumbnail to be semi-transparent and have a narrow red border. Since only one image will be selected at a time I decided to use an id called "current" for this purpose. The advantage of using CSS is that I can change how it will look at any time without changing the code. The style looks like this:
Here we see some code to handle browser differences again, the standard calls for using a opacity element, but both IE and Mozilla-based browsers don't support that yet.
Finally, I changed the HTML for the thumbnails to use the styles and call my function. The HTML for my slideshow now looks like this:
We haven't changed our JavaScript, so the slide show still works, but now we have more styling and our presentation is separated from our HTML and JavaScript. However, we have one problem, while the initially selected thumbnail is semi-transparent with a red border, it stays that way when we change images. To fix this, we need to turn back to our old friend JavaScript.
You can see a working example of the code so far here.
If you need a refresher (or first pass) at HTML and CSS, check out my favorite HTML and CSS book, HTML Dog: The Best-Practice Guide to XHTML and CSS and its accompanying website https://www.htmldog.com.
First I converted my existing styling to use CSS. If you aren't familiar with CSS, the easiest way to start experimenting with it is to put it between style tags in the head of your HTML document. The initial tag needs a type attribute to tell the browser what type of style information you are using, so it should look like this:
<style type="txt/css">
The initial conversion to CSS was easy since only the large image had styling information and it already had an id attribute that was being used for JavaScript purposes.
#largeImage {
border: 2px solid black;
width: 544px;
height: 408px;
}
I hadn't previously included sizing information for the thumbnails, but I added a class called thumbs and set image tags in that class to set the size to 40px by 40px. This means that even if I accidentally load images that are too large or small for thumbnails, they will be forced to display in thumbnail size.
img.thumbs{
border: none;
width: 40px;
height: 40px;
}
I also added a slideshow class to hold the entire slideshow. This will allow me to do things like add a border or change the background color for the whole slideshow if I desire. At this point I'm only using it to set the maximum height to the the height of the large image, because when I add more thumbnails I want them to stay on the side of the large image instead of moving above it. Unfortunately, Internet Explorer doesn't support the max-height attribute, so I'll still have to work on this issue more later.
.slideshow{
height: 408px;
max-height: 408px;
}
Finally I created a style for the selected thumbnail. I decided that I wanted my selected thumbnail to be semi-transparent and have a narrow red border. Since only one image will be selected at a time I decided to use an id called "current" for this purpose. The advantage of using CSS is that I can change how it will look at any time without changing the code. The style looks like this:
img#current{
border: 1px solid red;
filter:alpha(opacity=50);
-moz-opacity:0.5;
opacity: 0.5;
}
Here we see some code to handle browser differences again, the standard calls for using a opacity element, but both IE and Mozilla-based browsers don't support that yet.
Finally, I changed the HTML for the thumbnails to use the styles and call my function. The HTML for my slideshow now looks like this:
<div class="slideshow">
<span class="thumbs">
<img class="thumbs" id="current" src="simone_thumb.jpg" onClick='document.getElementById("largeImage").src="simone.jpg"' alt="Thumbnail of Dog with EARTH Sticker"/>
<img class="thumbs" id="julie" src="julie_thumb.jpg" onClick='document.getElementById("largeImage").src="julie.jpg"' alt="Thumbnail of laptop with EARTH Sticker"/>
</span>
<img id="largeImage" src="simone.jpg" alt="Larger verson of selected thumbnail"/>
</div>
We haven't changed our JavaScript, so the slide show still works, but now we have more styling and our presentation is separated from our HTML and JavaScript. However, we have one problem, while the initially selected thumbnail is semi-transparent with a red border, it stays that way when we change images. To fix this, we need to turn back to our old friend JavaScript.
You can see a working example of the code so far here.
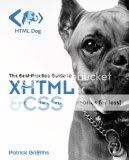
This site needs an editor - click to learn more!
You Should Also Read:
Minimalist JavaScript Slide Show
JavaScript Resources
Getting Ready to Learn JavaScript
Related Articles
Editor's Picks Articles
Top Ten Articles
Previous Features
Site Map
Content copyright © 2023 by Julie L Baumler. All rights reserved.
This content was written by Julie L Baumler. If you wish to use this content in any manner, you need written permission. Contact
BellaOnline Administration
for details.