JavaScript Slide Show With Functions

In previous articles we created and did some fine tuning on a very simple JavaScript slide show. This slide show is completely functional, and even displays something appropriate for people who don't have JavaScript, but I'd like to have the thumbnail of the image I'm currently displaying look different from the other thumbnails. I had settled on using a CSS id called "current" which made images semi-transparent and added a thin red border for the selected thumbnail. In my last article, I'd gotten this behavior for the initially selected thumbnail but when I selected a new thumbnail, the new thumbnail did not change and the initial thumbnail kept the selected styling.
In the previous version of my slide show, when a user clicks on a thumbnail, the associated large images is displayed. What I want it to do is change the large image, set the thumbnail of the previously selected image to go back to normal and make this thumbnail display with the special style for the selected image. Since I am now doing multiple things when the thumbnail is clicked on, I switched from putting all my code in the onClick attribute of the img tag to using a function. JavaScript functions usually go in the head section of the HTML so that they will be loaded and ready when the body of the page is loaded. They can either be inserted directly between script tags or put and a file and included. For short programs, or when I'm actively coding, I find it easier to put my code directly in the page as I did here.
I could have used a function for each thumbnail but since all that changes from thumbnail to thumbnail is the name of the image and the id of the thumbnail (and I used the image name for the id), I wrote a single function that took the id as an argument and used that to create the appropriate image name by adding ".jpg" to it. I called my function displayLarge.
In order for this function to work the first time, I also had to give an initial definition for oldID, the variable I am using to remember the original id of the thumbnail that is currently selected. All this code goes in the head section of the web page, so that it is loaded before the page loads.
Finally, I changed the HTML for the thumbnails to call my function. The HTML for my slideshow now looks like this:
You can see a working example of this code here.
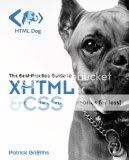
If you need a refresher (or first pass) at HTML and CSS, check out my favorite HTML and CSS book, HTML Dog: The Best-Practice Guide to XHTML and CSS and its accompanying website https://www.htmldog.com.

In the previous version of my slide show, when a user clicks on a thumbnail, the associated large images is displayed. What I want it to do is change the large image, set the thumbnail of the previously selected image to go back to normal and make this thumbnail display with the special style for the selected image. Since I am now doing multiple things when the thumbnail is clicked on, I switched from putting all my code in the onClick attribute of the img tag to using a function. JavaScript functions usually go in the head section of the HTML so that they will be loaded and ready when the body of the page is loaded. They can either be inserted directly between script tags or put and a file and included. For short programs, or when I'm actively coding, I find it easier to put my code directly in the page as I did here.
I could have used a function for each thumbnail but since all that changes from thumbnail to thumbnail is the name of the image and the id of the thumbnail (and I used the image name for the id), I wrote a single function that took the id as an argument and used that to create the appropriate image name by adding ".jpg" to it. I called my function displayLarge.
function displayLarge(id){
// change the large image
imageName=id + ".jpg";
document.getElementById("largeImage").src=imageName;
// set the style of the previous thumbnail back to the default
document.getElementById("current").id=oldID;
// make note of the id before we change it
oldID=id
// mark the thumbnail as current with the current style
document.getElementById(id).id="current";
}
In order for this function to work the first time, I also had to give an initial definition for oldID, the variable I am using to remember the original id of the thumbnail that is currently selected. All this code goes in the head section of the web page, so that it is loaded before the page loads.
Finally, I changed the HTML for the thumbnails to call my function. The HTML for my slideshow now looks like this:
<div class="slideshow">
<span class="thumbs">
<img class="thumbs" id="current" src="simone_thumb.jpg" onClick='displayLarge("s
imone")' alt="Thumbnail of Dog with EARTH Sticker"/>
<img class="thumbs" id="julie" src="julie_thumb.jpg" onClick='displayLarge("julie")' alt="Thumbnail of laptop with EARTH Sticker"/>
</span>
<img id="largeImage" src="simone.jpg" alt="Larger verson of selected thumbnail"/>
</div>
You can see a working example of this code here.
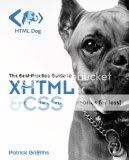
If you need a refresher (or first pass) at HTML and CSS, check out my favorite HTML and CSS book, HTML Dog: The Best-Practice Guide to XHTML and CSS and its accompanying website https://www.htmldog.com.
This site needs an editor - click to learn more!
You Should Also Read:
Minimalist JavaScript Slide Show
JavaScript Slide Show with CSS
Related Articles
Editor's Picks Articles
Top Ten Articles
Previous Features
Site Map
Content copyright © 2023 by Julie L Baumler. All rights reserved.
This content was written by Julie L Baumler. If you wish to use this content in any manner, you need written permission. Contact
BellaOnline Administration
for details.